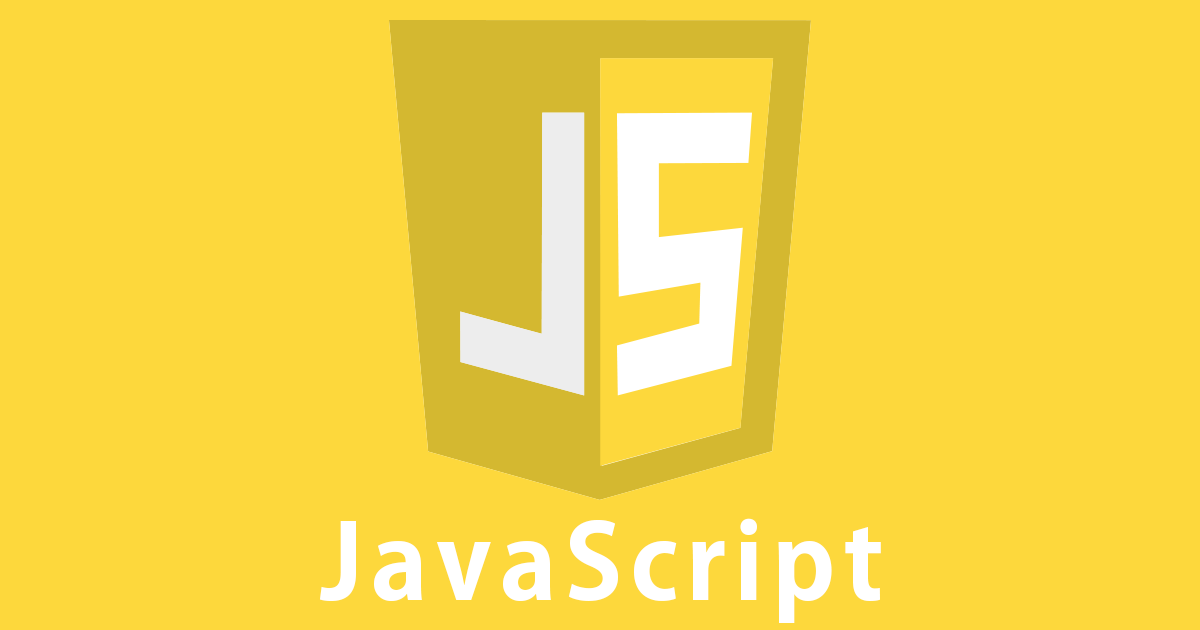
webpack-dev-server+devcertでhttpsなローカルサーバを起動する
吉川@京都です(実家に帰省中)。
viteでhttpsなlocalhostを起動する(devcert編) | DevelopersIO
先日こちらでdevcertを紹介しました。記事でも言っているように、これを使うメリットは
- 依存をNode.jsランタイムにまとめられる
- 証明書発行を自動化できる
になるかと思っています。
で、今回はviteではなくwebpackならどのような設定になるか、やってみたので共有します。
サンプルプロジェクトの作成
こちらを参考にミニマムなサンプルプロジェクトを作成します。
依存関係インストール
npm i lodash npm i -D webpack webpack-cli webpack-dev-server html-webpack-plugin
ディレクトリ構成
. ├── index.html ├── package.json ├── src │ └── index.js └── webpack.config.js
<!-- index.html --> <!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <title>Getting Started</title> </head> <body> <script src="main.js"></script> </body> </html>
// src/index.js import _ from 'lodash' function component() { const element = document.createElement('div') element.innerHTML = _.join(['Hello', 'webpack'], ' ') return element } document.body.appendChild(component())
// webpack.config.js const path = require('path') const HtmlWebpackPlugin = require('html-webpack-plugin') module.exports = { mode: 'development', entry: './src/index.js', devServer: { static: './dist', }, plugins: [ new HtmlWebpackPlugin({ title: 'Development', }), ], output: { filename: 'main.js', path: path.resolve(__dirname, 'dist'), clean: true, }, }
動作確認
npx webpack serve --open
httpで「Hello webpack」ページを返すローカルサーバを起動します。
devcert導入
webpack.config.jsを変更
では、devcertを導入してhttpsにします。
npm i -D devcert
devcert.certificateFor()
はPromiseを返すので、async/awaitをwebpack.config.jsで書きたいところです。
こちらを見た感じ return new Promise()
の書き方はできるようなので、同じ要領でasync/awaitで書けるか試してみたところ、意図通り動きました。
const path = require('path') const HtmlWebpackPlugin = require('html-webpack-plugin') const devcert = require('devcert') module.exports = async () => { // async関数にする const { key, cert } = await devcert.certificateFor('localhost') // 証明書発行 return { mode: 'development', entry: './src/index.js', devServer: { static: './dist', https: { // httpsを有効に key, // 証明書設定 cert, // 証明書設定 }, }, plugins: [ new HtmlWebpackPlugin({ title: 'Development', }), ], output: { filename: 'main.js', path: path.resolve(__dirname, 'dist'), clean: true, }, } }
動作確認
npx webpack serve --open
httpsでローカルサーバを起動できました。